Getting Started
How to get started using the BigMailer API
Creating an API key
An API key can be created by visiting the API key management page in the BigMailer console. On that page, click "Create Key" and enter a name. Choose a name that will help you remember what application is using the API key. Click "Create" in the dialog box. Copy the API key value. You will need it to make API calls.
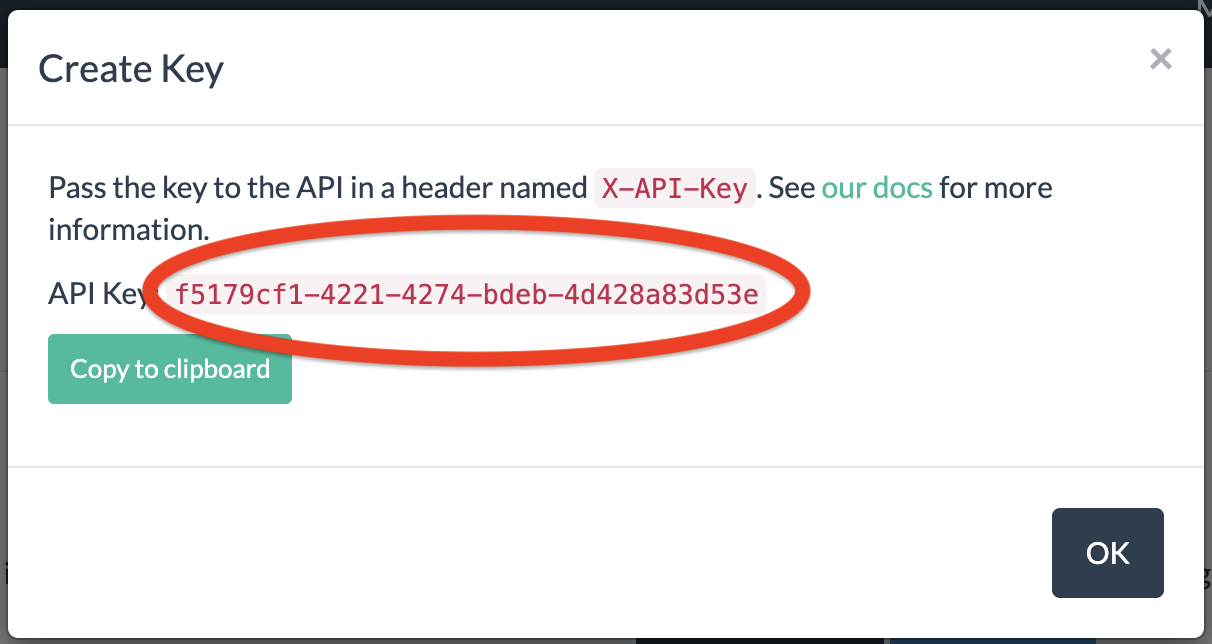
Making API Calls
API calls are made by executing HTTPS requests to the BigMailer API. The URL of all API endpoints begin with the prefix https://api.bigmailer.io/v1
. The HTTP header X-API-Key
must be specified on all API calls. The value of the X-API-Key
header must be an API key obtained from the API key management page. Request bodies of POST
and PUT
requests must be in JSON format.
Further information on performing specific API calls is found in the API Reference.
Rate Limiting
API calls are subject to per account rate limiting. By default accounts can perform 10 API calls per second. Additionally, accounts are limited to making 4 API calls concurrently. When either of these limits is exceeded, the API returns the HTTP status 429 Too Many Requests
.
Please contact us if your application requires more capacity than the default rate limits allow.
Sample API Call
You can check your application is properly configured by making an API call to https://api.bigmailer.io/v1/me
. Be sure to replace the sample API key shown below with your actual API key.
curl --request GET \
--url 'https://api.bigmailer.io/v1/me' \
--header 'Accept: application/json' \
--header 'X-API-Key: f5179cf1-4221-4274-bdeb-4d428a83d53e'
const fetch = require('node-fetch');
let url = 'https://api.bigmailer.io/v1/me';
let options = {
method: 'GET',
qs: {limit: '10'},
headers: {
Accept: 'application/json',
'X-API-Key': 'f5179cf1-4221-4274-bdeb-4d428a83d53e'
}
};
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://api.bigmailer.io/v1/me")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Get.new(url)
request["Accept"] = 'application/json'
request["X-API-Key"] = 'f5179cf1-4221-4274-bdeb-4d428a83d53e'
response = http.request(request)
puts response.read_body
import requests
url = "https://api.bigmailer.io/v1/me"
querystring = {"limit":"10"}
headers = {
"Accept": "application/json",
"X-API-Key": "f5179cf1-4221-4274-bdeb-4d428a83d53e"
}
response = requests.request("GET", url, headers=headers, params=querystring)
print(response.text)
<?php
$curl = curl_init();
curl_setopt_array($curl, [
CURLOPT_URL => "https://api.bigmailer.io/v1/me",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => [
"Accept: application/json",
"X-API-Key: f5179cf1-4221-4274-bdeb-4d428a83d53e"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Updated about 4 years ago